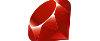
The default method to pass arguments to Rake tasks is to give the parameters in square brackets –
desc 'Method #1: Use the default rake way to add two numbers and log the result' task :add, [:num1, :num] do |t, args| puts args[:num1].to_i + args[:num].to_i end
Reference: 4 ways to pass arguments to a Rake task
$ rake add[1,2] # => 3
However, I would rather pass the arguments like this –
$ rake add 1 2 # => 3
This is Method #3, in the article above.
The key part here is that all the arguments passed should be symbolized. The rake task should then be –
desc 'Method #3: Use ARGV to add two numbers and log the result' task :add do ARGV.each { |a| task a.to_sym do ; end } puts ARGV[1].to_i + ARGV[2].to_i end
For whatever reason, if the arguments don’t get symbolized, we get this error
$ rake add 1 2 ["add", "1", "2"] 3 rake aborted! Don't know how to build task '1' (See the list of available tasks with `rake --tasks`)
So, the way to avoid this error, even when the arguments are not symbolized, is to add exit!
at the end of the rake task –
desc 'Method #3: Use ARGV to add two numbers and log the result' task :add do puts ARGV[1].to_i + ARGV[2].to_i exit! end