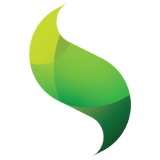
ExtJs, by default, doesn’t provide a month picker.
A monthfield picker allows you to choose specific months only.
The customization that was required, was to have a start date and an end date for those months, so that at the end of the operation, those specific dates could be saved.
The MonthField field is derived from the Ext.form.field.Date with the picker completely customized to show months instead of the days of the calendar.
Ext.define('Ext.form.field.Month', { extend: 'Ext.form.field.Date' , alias: 'widget.monthfield' , requires: ['Ext.picker.Month'] , lastDay: false , selectMonth: null , createPicker: function () { var me = this , format = Ext.String.format ; return Ext.create('Ext.picker.Month', { pickerField: me , ownerCt: me.ownerCt , renderTo: document.body , floating: true , hidden: true , focusOnShow: true , minDate: me.minValue , maxDate: me.maxValue , disabledDatesRE: me.disabledDatesRE , disabledDatesText: me.disabledDatesText , disabledDays: me.disabledDays , disabledDaysText: me.disabledDaysText , format: me.format , showToday: me.showToday , startDay: me.startDay , minText: format(me.minText, me.formatDate(me.minValue)) , maxText: format(me.maxText, me.formatDate(me.maxValue)) , listeners: { select: { scope: me, fn: me.onSelect } , monthdblclick: { scope: me, fn: me.onOKClick } , yeardblclick: { scope: me, fn: me.onOKClick } , OkClick: { scope: me, fn: me.onOKClick } , CancelClick: { scope: me, fn: me.onCancelClick } } , keyNavConfig: { esc: function () { me.collapse(); } } }); } , onCancelClick: function () { this.selectMonth = null; this.collapse(); } , onOKClick: function () { if (this.selectMonth) { this.setValue(this.selectMonth); this.fireEvent('select', this, this.selectMonth); } this.collapse(); } , onSelect: function (m, d) { var date , month = d[0] + 1 , year = d[1] ; if (this.lastDay) { date = Ext.Date.getLastDateOfMonth(new Date(month + '/01/' + year)); var checkMonth = date.getMonth() + 1; if (month < checkMonth) { var prevMonth = month - 1; date = Ext.Date.getLastDateOfMonth(new Date(prevMonth + '/01/' + year)); } } else { date = new Date(month + '/01/' + year); } this.selectMonth = date; } });
Here, a widget of xtype monthfield is created.
The monthfield picker can then be embedded into a form.
Ext.define('App.view.Main', { extend: 'Ext.panel.Panel' , viewModel: { data: { current: new Date() } , formulas: { startDate: function (get) { var current = get('current'); return Ext.Date.getFirstDateOfMonth(current); } , endDate: function (get) { var current = get('current'); return Ext.Date.getLastDateOfMonth(new Date); } } } , title: 'Monthfield Picker' , titleAlign: 'center' , bodyPadding: 20 , defaults: { xtype: 'monthfield' , format: 'd M Y' , margin: 20 } , items: [{ fieldLabel: 'Start Date' , bind: { value: '{startDate}' } , listeners: { } }, { fieldLabel: 'End Date' , lastDay: true , bind: { value: '{endDate}' } }] });
Here, using the viewModel formulas, we set the first and the last date of the month