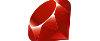
There have been many times over the years that I’ve been wanting a simple capability of monitoring changes in some directory or some files in my system.
Linux has inotify and Windows has WatchDirectory.
The biggest challenge has been to look for something that works seamlessly across operating systems.
So, I thought of creating my own solution!
And as usual, I looked at Ruby first since that is, by default, on every system I touch!
Although, in my opinion, this program could as well have been written in any language.
The idea I came up with was
- get modified times of all files (even nested) inside a directory
- save them as a string to a file
- compare this every interval
The program eventually turned out looking like this –
prev = File.read('previous_times.txt') rescue "" watch_directory = File.expand_path(File.join('directory/to/monitor', '**/*')) mtimes = Dir[watch_directory].collect do |file_name| File.mtime file_name if File.file? file_name end.to_s puts "=== Have files been modified? ===" p mtimes != prev File.write('previous_times.txt', mtimes)
And to run this every 10 seconds, it can be wrapped in a while loop with a sleep.
while true ... # above code ... sleep 10 end
As usual, in situations like these, the best test is to check against a node_modules
folder. So, I tried it on one which had 9,379,193 files and which took around 75 seconds to scan. And it worked perfectly there too!