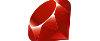
After having created the directory monitor, I realized that it would not be suitable for everything.
For example, if I needed to know which file was getting modified in the directory, rather than just anything having gotten modified.
Well, in that case we would somehow need to store both the file name and the modified time of the file which changed.
I thought of using some file to store the information in and then read it from there. But instead, I decided to use yaml/store
which is a very interesting library in it’s own right.
require 'yaml/store' watch_directory = File.expand_path(File.join('directory/to/monitor', '**/*')) db = YAML::Store.new('previous_times.yaml') prev = [] db.transaction do prev = db['mtimes'].nil? ? [] : db['mtimes'].collect { |r| r } end mtimes = Dir[watch_directory].collect do |file_path| if File.file? file_path file_name = File.basename file_path { file: file_name, mtime: File.mtime(file_path) } end end if prev != mtimes and !prev.empty? puts " === files modified ===" diff_elements = mtimes - prev diff_elements.each do |r| p r[:file] end end db.transaction do db['mtimes'] = mtimes end
This would give an output like this
=== files modified === file1.txt
And, as usual, to run this every 10 seconds, it can be wrapped in a similar while loop with a sleep.
while true ... # above code ... sleep 10 end